Filter
Filters can be added in AngularJS to format data to display on UI without changing the original format. Filters can be added to an expression or directives using the pipe (|) symbol.
Syntax
{{expression | filterName:parameter }}
AngularJS Filters:AngularJS provides filters to transform data of different data types. The following table shows the significant filters:
- currency:It is used to convert a number into a currency format.
- date:It is used to convert a date into a specified format.
- filter:It is used to filter the array and object elements and return the filtered items.
- lowercase:It is used to convert a string into lowercase letters.
- uppercase:It is used to convert a string into uppercase letters.
- number:It is used to convert a number into a string or text.
- orderBy:It sorts an array based on specified predicate expressions.
4.1 Built-in filters-
1. Upper case
The uppercase Filter in AngularJS is used to change a string to uppercase string or letters.
Syntax:
{{string | uppercase}}
Example:
<html><head>
<title> uppercase Filter </title>
<script src="angular.min.js"></script>
</head>
<body ng-app="app">
<h2>uppercase Filter </h2>
<div ng-controller="upper">
<p>
<span style="color:green">
{{msg | uppercase}}
</span> used to change format of text into uppercase.
</p>
</div>
<script>
angular.module('app', []).controller('upper', ['$scope',
function($scope) {
$scope.msg = 'Uppercase filter';
}]);
</script>
</body>
</html>
2. Lower case
The lowercase filter is used to convert a string into lowercase letters.
Syntax:
{{expression|lowercase}}
Example
<html>
<script src="angular.min.js"></script>
<body>
<h2>AngularJS - lowercase</h2>
<div ng-app="myApp" ng-controller="myCtrl">
<strong>Input:</strong><input type="text" ng-model="string">
<strong>Output:</strong>{{string | lowercase}}
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.string = "";
});
</script>
</body>
</html>
3. date
AngularJS date filter is used to convert a date into a specified format. When the date format is not specified, the default date format is 'MMM d, yyyy'.
Syntax:
{{ date | date : format : timezone }}
<html>
<head>
<script src="angular.min.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="dateCntrl">
<p>{{ today | date : "dd.MM.y" }}</p>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('dateCntrl', function($scope) {
$scope.today = new Date();
});
</script>
</body>
</html>
4. currency
AngularJS currency filter is used to convert a number into a currency format. If no currency format is specified currency filter uses the local currency format.
Syntax:
{{ currency_expression | currency : symbol : fractionSize}}
Parameters:It contains 2 parameters as mentioned above and described below:
- symbol:It is an optional parameter. It is used to specify the currency symbol. The currency symbol can be any character or text.
- fractionsize:It is an optional parameter. It is used to specify the number of decimals.
<html>
<head>
<script src="angular.min.js"> </script>
</head>
<body>
<h3>AngularJS currency Filter</h3>
<div ng-app="myApp" ng-controller="currencyCntrl">
<h3>
Currency filter with currency symbol and fraction size.
</h3>
<p>Amount : {{ amount | currency : "₹" :2}}</p>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('currencyCntrl', function ($scope) {
$scope.amount = 77.525;
});
</script>
</body>
</html>
5. Number formatting
AngularJS number filter is used to convert a number into a string or text. We can also define a limit to display a number of decimal digits. The number filter rounds off the number to specified decimal digits.
Syntax:
{{ string| number : fractionSize}}
Parameter Values:It contains single parameter value fractionSize which is of type number and used to specify the number of decimals.
Example
<html>
<head>
<script src="angular.min.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="numberCntrl">
<h3>Number filter with fraction size.</h3>
<p>Number : {{ value| number : 2}}</p>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('numberCntrl', function($scope) {
$scope.value = 84998.548;
});
</script>
</body>
</html>
6. orderBy
An orderBy Filter in AngularJS is used to sort the given array to the specific order. The default order of sorting the string is in alphabetical order whereas the numbers are numerically sorted. By default, all the items are sorted in ascending order, if the ordering sequence is not specified.
Syntax:
{{orderBy_expression | orderBy: expression: reverse: comparator}}
- expression:This parameter value can be used to determine the order while filtering the items. It can be the following types:
- reverse:It is an optional parameter that will be required to reverse the order of the array if its value is set to true.
<html>
<head> <script src="angular.min.js"></script></head>
<body>
<div ng-app="myApp" ng-controller="orderCtrl">
<h3>AngularJS orderBy Filter</h3>
<ul>
<li ng-repeat="x in customers | orderBy : 'name'">
{{x.name + ", " + x.city}}
</li>
</ul>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('orderCtrl', function($scope) {
$scope.customers = [{
"name": "Amber",
"city": "ajmer"
},
{
"name": "lakshay",
"city": "vizag"
},
{
"name": "karan",
"city": "London"
},
{
"name": "bhaskar",
"city": "peshawar"
}, ];
});
</script>
<p>The array items are arranged by "name".</p>
</body>
</html>
7. Filter
In other words, this filter selects a subset (a smaller array containing elements that meet the filter criteria) of an array from the original array.
Syntax:
{{arrayexpression | filter: expression : comparator : anyPropertyKey}}
- arrayexpression:The source array on which the filter will be applied.
- expression:It is used to select the items from the array after the filter conditions are met. It can be of String type, Function type, or Object type.
- comparator: It is used to determine the value by comparing the expected value from the filter expression, and the actual value from the object array.
- anyPropertyKey:It is an optional parameter having the special property that is used to match the value against the given property. It is of String type & its default value is $.
Example:
<html><head><script src="angular.min.js"></script></head>
<body>
<div ng-app="myApp" ng-controller="namesCtrl">
<h3>AngularJS filter Filter</h3>
<ol>
<strong><li ng-repeat="x in names | filter : 'e'">
{{ x }}
</li>
</strong>
</ol>
</div>
<script>
angular.module('myApp', []).controller('namesCtrl', function($scope) {
$scope.names = ['Jani','Carl', 'Margareth','Hege Mathew', 'Joey ];
});
</script>
</body>
</html>
8. Custom filter
- Sometimes the built-in filters in Angular cannot meet the needs or requirements for filtering output. In such a case, an AngularJS custom filter can be created, which can pass the output in the required manner.
- To crate it the same way we have created controllers & modules.
- With the help of angular.filter we can create custom filter.
Syntax:
app.filter( filtername, function()
{ return function (input, value1,value2)
{ var input; return output;}
}
);
Example
<html><head>
<title>AngularJS filter Filter</title>
<script src="angular.min.js"></script>
<script type="text/javascript">
var app=angular.module('myapp',[]);
app.filter('convertUppercase',function()
{ return function(item)
{return item.toUppercase();
};
});
app.controller('Custromctrl',function($scope)
{
$scope.name='angularJS';
});
</script>
</head>
<body ng-app="myapp">
<div ng-controller="CustomCtrl">
<p>
{{name |convertUppercase}}
</p>
</div>
</body>
</html>
4.2 Angular JS Forms
Working with AngularJS forms
- AngularJS facilitates you to create a form enriches with data binding and validation of input controls.
- Input controls are ways for a user to enter data. A form is a collection of controls for the purpose of grouping related data together.
- Following are the input controls used in AngularJS forms:
- input elements
- select elements
- button elements
- textarea elements
- In AngularJS data binding to input controls can be done by using ng-model directive.
- The ng-model directive in AngularJS used to bind & get data from input controls & it will provide two way data binding by synchronizing data between model to view & vice-versa.
Example
<html><head>
<title>AngularJS Form Example</title>
<script src="angular.min.js">
</script>
<script type="text/javascript">
var app=angular.module('myapp',[]);
app.controller('formctrl',function($scope)
{
$scope.pname="sachin";
$scope.gname="cricket";
});
</script>
</head>
<body >
<div ng-app="myapp" ng-controller="formctrl'">
Player's Name:<input type="text" ng-model="pname"/><br>
Game Name:<input type="text" ng-model="gname"/><br>
<p> Detail Information: {{pname+" "+gname}}</p>
</div>
</body>
</html>
Model binding
Using ng-model directives as an attribute we will bind data to input controls.
Example
<html><head>
<title>AngularJS Form Example</title>
<script src="angular.min.js">
</script>
<script type=”text/javascript”>
var app=angular.module('myapp',[]);
app.controller('formctrl',function($scope)
{
$scope.pname=”sachin”;
$scope.gname=”cricket”;
$scope.getvalues=function()
{
$scope.name = $scope.pname+ “ “+$scope.gname;
}
});
</script>
</head>
<body >
<div ng-app="myapp" ng-controller="formctrl'">
Player's Name:<input type=”text” ng-model="pname"/><br>
Game Name:<input type="text" ng-model="gname"/><br>
<input type="button" value="SeeDetails" ng-click="getvalues()"/>
<b> Detail Information: {{ name}}</b>
</div>
</body>
</html>
Custom validation
- AngularJS allows us to create our own validation function.
- We have to add new directive to our application and deal with validation inside function with certain arguments.
Example:
<html><head>
<title>AngularJS Form Validation</title>
<script src="angular.min.js">
</script>
</head>
<body ng-app="developapp">
<h3>AngularJS Custom Form Validation</h3>
<form name="form1">
Username:<input name="username" required><br>
Age:<input name="userage" ng-model="userage" required app-directive>
</form>
<p>The input's valid state is:</p>
<h3>{{form1.userage.$valid}}</h3>
<script>
var app = angular.module('developapp', []);
app.directive('appDirective', function () {
return {
require: 'ngModel',
link: function (scope, element, attr, mCtrl) {
function myValidation(value) {
if (value >= 18) {
mCtrl.$setValidity('charE', true);
} else {
mCtrl.$setValidity('charE', false);
}
return value;
}
mCtrl.$parsers.push(myValidation);
}
};
});
</script>
<p>
<b>Note:</b>
The input field must have user age greater than 18 to be considered valid for voting.
</p>
</body>
</html>
Form Events:
An Events in AngularJS can be used to perform particular tasks, based on the action taken. Both Angular Event & the HTML Event will be executed & will not overwrite with an HTML Event. It can be added using the Directives mentioned below:
- ng-mousemove:The movement of the mouse leads to the execution of the event.
- ng-mouseup:Movement of the mouse upwards leads to the execution of the event.
- ng-mousedown:Movement of the mouse downwards leads to the execution of the event.
- ng-mouseenter:Click of the mouse button leads to the execution of the event.
- ng-mouseover:Hovering the mouse leads to the execution of the event.
- ng-cut:Cut operation leads to the execution of the event.
- ng-copy:Copy operation leads to the execution of the event.
- ng-keypress:Press of key leads to the execution of the event.
- ng-keyup:Press of upward arrow key leads to the execution of the event.
- ng-keydown:Press of downward arrow key leads to the execution of the event.
- ng-click:Single click leads to the execution of the event.
- ng-dblclick: Double click leads to the execution of the event.
- ng-blur:Fired when an HTML element loses its focus.
- ng-focus:It is used to apply custom behavior when an element is focused.
- ng-paste:It is used to specify custom behavior functions when the text in input fields is pasted into an HTML element.
- ng-mouseleave:It is used to apply custom behavior when a mouse-leave event occurs on a specific HTML element.
Example:
<html><head>
<script src="angular.min.js"> </script>
</head>
<body>
<p> Move the mouse over COUNT to increase the Total Count.</p>
<div ng-app="App1" ng-controller="Ctrl1">
<h1 ng-mousemove="count = count + 1"> COUNT </h1>
<h2>Total Count:</h2> <h2>{{ count }}</h2>
</div>
<script>
var app = angular.module('App1', []);
app.controller('Ctrl1', function($scope) {
$scope.count = 0;
});
</script>
</body>
</html>
AngularJS service
- AngularJS service are JavaScript function or object, who's responsible to perform specific tasks and can be reused throughout the application.
- It provide many inbuilt services. In AngularJS services starts with "$".
- Example: $http, $window, $location etc.
- These AngularJS services interact with controller, model or custom directives.
$http service
- It makes the request to the server, in order to handle the response by the application.
- The $http service is used to send or receive data from the remote server using browser's XMLHttpRequest or JSONP.
- $http is a service as an object. It includes following shortcut methods.
Method | Description |
---|---|
$http.get() | Perform Http GET request. |
$http.head() | Perform Http HEAD request. |
$http.post() | Perform Http POST request. |
$http.put() | Perform Http PUT request. |
$http.delete() | Perform Http DELETE request. |
$http.jsonp() | Perform Http JSONP request. |
$http.patch() | Perform Http PATCH request. |
$http.get()
- $http.get() method sends http GET request to the remote server and retrieves the data.
- Signature: HttpPromise $http.get(url)
- $http.get() method returns HttpPromise object, which includes various methods to process the response of http GET request.
- The following example demonstrates the use of $http service in a controller to send HTTP GET request.
Example:
<html><head> <script src="angular.min.js"> </script></head>
<body ng-app ="myApp">
<div>
<div ng-controller="myController">Response Data: {{data}} <br/>
Error: {{error}}
</div>
</div>
<script>
var myApp = angular. module ('myApp', []);
myApp.controller ("myController", function ($scope, $http) {
var onSuccess = function (data, status, headers, config) {
$scope.data = data; };
var onError = function (data, status, headers, config) {
$scope.error = status; }
var promise = $http.get("/demo/getdata");
promise.success (onSuccess);
promise.error (onError); });
</script>
</body>
</html>
4.3 Ajax implementation using $http
- AJAX is an acronym which stands for Asynchronous JavaScript and XML.
- It is important tool in any web application. It is a group of inter-related technologies like JavaScript, DOM, XML, HTML/XHTML, CSS, and XMLHttpRequest.
- As name indicate it performs asynchronous activity that will increase the performance. To enhance the performance of our application we can use Ajax in AngularJS application.
- AJAX allow us to send & receive data asynchronously without reloading the web page. So it is fast
How Ajax Work
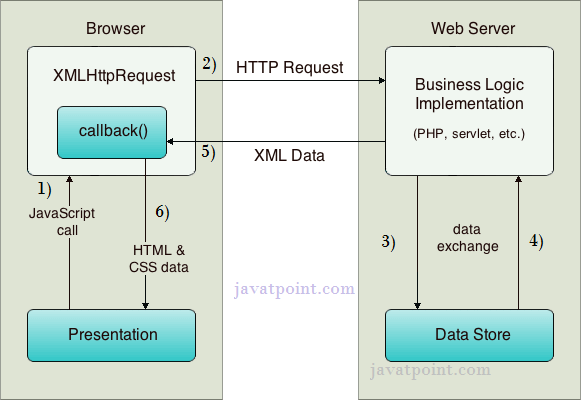
As you can see in the above example, XMLHttpRequest object plays a important role.
- User sends a request from the UI and a javascript call goes to XMLHttpRequest object.
- HTTP Request is sent to the server by XMLHttpRequest object.
- Server interacts with the database using JSP, PHP, Servlet, ASP.net etc.
- Data is retrieved.
- Server sends XML data or JSON data to the XMLHttpRequest callback function.
- HTML and CSS data is displayed on the browser.
- AngularJS provide $http service to read data from the server.
- The server make a database call to get the. desired records or data
- AngularJS need data in JSON (JavaScript Object Notation) format.
- Once the data is ready, $http can be used to get the data from server.
JSON file format
[{ "Name":"Sagar Ghathkar",
"RollNo":"101",
"Percentage":"60%"
}
{ "Name”:"Sachin Thakare",
"RollNo":"102",
"Percentage":"65%"
},
]
Example of $http service in controller
function studentsController($scope, $https:){
Var url="demo.txt";
$https: .get (url).success (function (response)
{
$scope. Student=response;
});
}
Code for Ajax implementation using $http
Example:
<html><head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js"> </script>
</head>
<body ng-app ="myApp">
<h2> AngularJS Ajax Implementation Application</h2>
<div ng-app="" ng-controller="studentController">
<table border=2>
<tr>
<th>Name</th>
<th>Roll No</th>
<th>Percentage</th>
</tr>
<tr ng-repeat="Student in students">
<td>{{student.Name}}</td>
<td>{{student.RollNo}}</td>
<td>{{student.Percentage}}</td>
</tr>
</table>
</div>
<script>
function studentsController ($scope, $https )
{
Var url=”demo.txt”;
$https.get (url).success (function (response)
{
$scope. Student=response;
});
}
</script>
</body>
</html>
No comments:
Post a Comment