ACTIVITY, INTENT AND LAYOUT
2.1 Introduction to Activity
- An activity is the single screen in android. It is like window or frame of Java.
- By the help of activity, we can place all our UI components or widgets in a single screen.
- Activity class is one of the very important parts of the Android Component. Any app, don't matter how small it is (in terms of code and scalability), has at least one Activity class.
- Unlike most programming languages, in which the main() method is the entry point for that program or application to start its execution, the android operating system initiates the code in an Activity instance by invoking specific callback methods its Lifecycle. So it can be said that An activity is the entry point for interacting with the user.
- Every activity contains the layout, which has a user interface to interact with the user.
2.2 Activity life cycle
- Android Activity Lifecycle is controlled by 7 methods of android.app.Activity class.
- The android Activity is the subclass of ContextThemeWrapper class.
- The 7 lifecycle method of Activity describes how activity will behave at different states.
Android Activity Lifecycle methods
Let's see the 7 lifecycle methods of android activity.
Method | Description |
---|---|
onCreate | called when activity is first created. |
onStart | called when activity is becoming visible to the user. |
onResume | called when activity will start interacting with the user. |
onPause | called when activity is not visible to the user. |
onStop | called when activity is no longer visible to the user. |
onRestart | called after your activity is stopped, prior to start. |
onDestroy | onDestroy called before the activity is destroyed. |

2.3 Introduction to Intent
- In Android, it is quite usual for users to witness a jump from one application to another as a part of the whole process, for example, searching for a location on the browser and witnessing a direct jump into Google Maps or receiving payment links in Messages Application (SMS) and on clicking jumping to PayPal or GPay (Google Pay).
- This process of taking users from one application to another is achieved by passing the Intent to the system.
- Android Intent is the message that is passed between components such as activities, content providers, broadcast receivers, services etc.
Below are some applications of Intents:
- Sending the User to Another App
- Getting a Result from an Activity
- Allowing Other Apps to Start Your Activity
- Display a web page
- Display a list of contacts
- Broadcast a message
- Dial a phone call etc.
2.4 Types of Intent
(1) Implicit Intent
Implicit Intent doesn't specifiy the component. In such case, intent provides information of available
components provided by the system that is to be invoked.
For example, you may write the following code to view the webpage.
-
Intent intent=new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse("http://www.google.com"));
startActivity(intent);
(2) Explicit Intent
Explicit Intent specifies the component. In such case, intent provides the external class to be invoked.
-
Intent i = new Intent(getApplicationContext(), ActivityTwo.class);
startActivity(i);
2.5 Layout Manager
Adapters are only responsible for creating and managing views for items (called ViewHolder), these classes do not decide how these views are arranged when displaying them. Instead, they depend on a separate class called LayoutManager.
LayoutManager is a class that tells Adapters how to arrange those items. For example, you might want those items in a single row top to bottom or you may want them arranged in Grids like Gallery. Instead of writing this logic in your adapter, you write it in LayoutManager and pass that LayoutManager to View (RecyclerView).
2.5.1 View and View Group
- View is the basic building block of UI(User Interface) in android.
- View refers to the android.view.View class, which is the super class for all the GUI components like TextView, ImageView, Button etc.
- View can be considered as a rectangle on the screen that shows some type of content. It can be an image, a piece of text, a button or anything that an android application can display.
- The rectangle here is actually invisible, but every view occupies a rectangle shape. The question that might be bothering you would be, what can be the size of this rectangle?
- The answer is either we can set it manually, by specifying the exact size (with proper units) or by using some predefined values.
- These predefined values are match_parent and wrap_content.
match_parent means it will occupy the complete space available on the display of the device.
wrap_content means it will occupy only that much space as required for its content to display.

A View is also known as Widget in Android. Any visual(that we can see on screen) and interactive(with which user can interact with) is called a Widget.
ViewGroup
- A ViewGroup is a special view that can contain other views.
- The ViewGroup is the base class for Layouts in android,like LinearLayout, RelativeLayout, FrameLayout etc.
- In other words, ViewGroup is generally used to define the layout in which views (widgets) will be set/arranged/listed on the android screen.
- ViewGroups acts as an invisible container in which other Views and Layouts are placed.
- Yes, a layout can hold another layout in it, or in other words a ViewGroup can have another ViewGroup in it.
- The class ViewGroup extends the class View.
2.5.2 Linear Layout
In a vertical LinearLayout, as the name suggests, the Views defined inside the Linear Layout are arranged verically one after another, like in a column. And for this we need to mention the android:orientation attribute with value vertical within the LinearLayout tag.
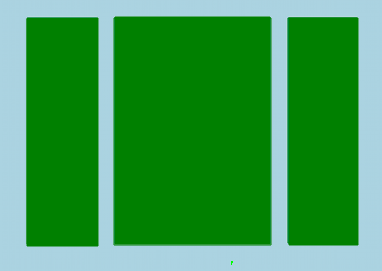
2.5.3 Relative Layout
It is flexible than other native layouts as it lets us to define the position of each child View relative to the other views and the dimensions of the screen.
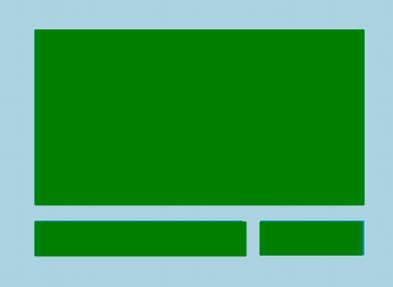
2.5.4 Table Layout
A TableLayout is a ViewGroup that arranges its children i.e Views and other Layouts in a table form with
rows and columns. To define a row, you can use
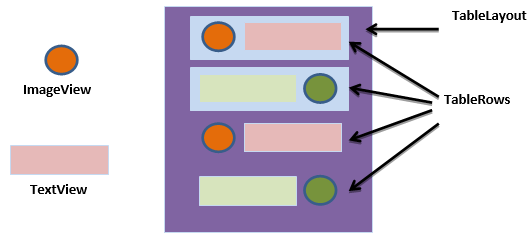
2.5.5 Grid Layout
A layout that places its children in a rectangular grid. The grid items are not predetermined but they automatically inserted to the layout using a List Adapter. A GridView is a type of AdapterView that displays items in a two-dimensional scrolling grid. Items are inserted into this grid layout from a database or from an array.
XML attributes of GridView
Android:numColumns
Android:horizontalSpacing
Android:verticalSpacing
2.5.6 Constraint Layout
It is layout on android that gives you adaptable and flexible ways to create views for your apps.Default layout in android studio gives you many ways to place objects.
Advantage:
Improve the UI performance over other Layouts. We can control the group of widgets through a single line of code. We can easily add animations to the UI components which we used in our app.
2.5.7 Frame Layout
The Framelayout is a placholder on screen that we can use to display a single view. Views that we add to a Framelayout are always anchored to the top left of the layout.
2.5.8 Scroll Layout
It is a special type of the framelayout in that it enables users to scroll trough a list of views that occupy more space than physical display allows. The scrollview can contain one other child view and enables scrolling the child view.
No comments:
Post a Comment